Getting Started with JavaScript Promises
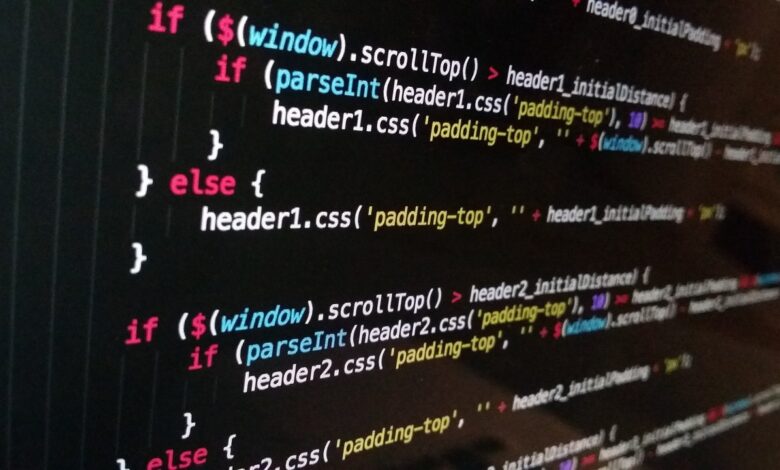
JavaScript promises are a fundamental concept in modern web development, providing a powerful way to manage asynchronous operations. They simplify code that involves tasks like fetching data, interacting with APIs, or performing time-sensitive computations, making it easier to handle success, failure, and sequencing. Understanding promises is key to writing clean, readable, and efficient JavaScript code.
At their core, promises represent a placeholder for a value that may not be available yet but will resolve at some point in the future. This value can be either the successful result of an operation or an error that occurred during its execution. Promises are an improvement over traditional callback-based programming, addressing issues like “callback hell,” where nested callbacks make the code difficult to read and maintain.
Anatomy of a Promise
A promise has three states:
- Pending: The initial state, where the promise neither has a result nor an error.
- Fulfilled: The promise has successfully completed, returning a result.
- Rejected: The promise has failed, returning an error.
Once a promise is fulfilled or rejected, it is considered “settled,” and its state cannot change.
Promises are created using the Promise
constructor. For example:
In this code, the resolve
function is called when the operation succeeds, and the reject
function is called in case of failure.
Handling Promises
To use a promise, you typically attach handlers using the .then()
and .catch()
methods. The .then()
method handles the resolved value, while .catch()
manages errors:
The beauty of promises lies in their ability to chain operations. When a .then()
handler returns another promise, the next .then()
in the chain waits for it to resolve:
This approach ensures that each step is executed in sequence and any errors in the chain are caught and handled gracefully.
Combining Promises
JavaScript provides methods like Promise.all
, Promise.race
, and Promise.allSettled
to work with multiple promises simultaneously:
Promise.all
: Resolves when all promises in the array are fulfilled or rejects when any promise fails.Promise.race
: Resolves or rejects as soon as the first promise settles.Promise.allSettled
: Waits for all promises to settle, providing their results or errors without short-circuiting.
These methods are particularly useful in scenarios where you need to wait for multiple asynchronous tasks to complete.
Async/Await: A Syntactic Enhancement
While promises are powerful, managing them can become verbose. The async/await
syntax, introduced in ES2017, simplifies working with promises by making asynchronous code look synchronous:
This approach enhances readability and reduces the cognitive load associated with chaining .then()
and .catch()
calls.
Conclusion
JavaScript promises are an essential tool for developers, enabling more organized and manageable asynchronous programming. By understanding their structure, handling mechanisms, and methods for combination, developers can write more efficient and maintainable code. With the additional support of async/await
, promises become even more accessible, helping developers build robust and scalable applications. Embracing promises is a step towards mastering modern JavaScript development.